
In this tutorial,
We will learn How to create a CSV text file (Comma-separated values ) using C# language on the dotnet platform (.NET Platform) and store data into the file. This tutorial is aimed at beginners and teaches you to create a CSV file, how to write the CSV headers to the text file and finally how to store data into the CSV file using C# language.
A Comma-Separated Values (CSV) text file is a simple format for storing and organizing data in a tabular structure using plain text. Each line in a CSV file represents a row of data, with values separated by commas. The first line typically contains headers that describe each column, such as "Name," "Age," and "City," while subsequent lines hold the corresponding data for each entry. CSV files are easy to read and can be opened with any text editor.
Contents
- IDE's Used
- Creating a CSV text File using C#
- Source Code for C# CSV file Creator
- Selecting the Delimiter for your CSV File
- Creating the header for your CSV file
- Adding Data to your CSV file
- Writing the data in CSV format using C#
- Reading Data from CSV file using C#
- Creating a TAB separated Values File in C#
IDE's Used
Here we will be using Microsoft Visual Studio to develop our project.
You can also use the Microsoft .NET SDK CLI tools for the same.
If you are new to Microsoft .NET SDK CLI tools,
Do Check out our introductory tutorial on using .NET SDK CLI tools here.
Creating a CSV text File using C#
Since CSV file is basically a text file, we will use the StreamWriter class available with the C# base library to create the text file.
StreamWriter is a class in C# used for writing text to a stream, such as a file. It’s part of the System.IO namespace and is particularly useful for creating and manipulating text files.The code below will create a simple text file using the StreamWriter class methods. We will modify this code to create a CSV file later.
//Create a CSV text file in your disk
using System;
using System.Text;
namespace CSV_TextFile_Read_Write
{
class CSVTextFileCreator
{
public static void Main()
{
try
{
// Using StreamWriter to create and write to a file
using (StreamWriter fwriter = new StreamWriter("CSVFileName.csv", true)) //true opens the file in append mode
{
fwriter.WriteLine("Text to be written to the file "); // write the header text to file
}
}
catch (Exception Ex)
{
Console.WriteLine($"An error occurred: {Ex.Message}");
}
}//end of main()
}//end of class
}//end of namespace
On running this code ,a text file is create on the disk with the name CSVFileName.CSV and some text is written into it .
In the below code snippet ,
using (StreamWriter fwriter = new StreamWriter("CSVFileName.csv", true)) //true opens the file in append mode
{
fwriter.WriteLine("Text to be written to the file "); // write the header text to file
}
We are creating a StreamWriter object called fwriter and initializing the constructor of the StreamWriter with the name of the csv text file (CSVFileName.csv) to be created and a Boolean value (true).This will create a file on the disk if it does not exist or open a connection to it if it is already exists.
The true parameter specifies that we are opening the file in append mode, so data will be added to the file instead of being overwritten.
here we use the fwriter.WriteLine() method to add text to the file.
The using statement ensures that the StreamWriter is disposed of correctly after use, which means the file is closed, and resources are released.
Source Code for C# CSV file Creator
Once we have learned how to create a text file ,We will now delve into the full source code of the CSV file creator code shown below.
GitHub Repo
Source code along with Visual studio Project files available on GitHub. Please use the Source codes from the Repo.
Browse CSV file Creation using C# code Repo.
The code below is only for demonstration purpose, some parts may be missing.
using System;
using System.Text;
namespace CSV_TextFile_Read_Write
{
class CSVTextFileCreator
{
public static void Main()
{
//CSVfile creation parameters
string SeperatorCharacter = ","; // Comma Seperated file
string CSVFileName = "CSVTextFile.csv"; // Name of the file to be created
string[] CSVHeaderTextArray = { "No", "Name", "Age", "Address" }; //CSV header data
//CSV data to be stored
string[,] CSVDataArray = {
{ "1", "Alice", "30", "123 Main St, New York" },
{ "2", "Bob", "25", "456 Elm St, Los Angeles" },
{ "3", "Charlie", "35", "789 Oak St, Chicago" },
{ "4", "David", "40", "321 Pine St, Houston" },
{ "5", "Eve", "28", "654 Maple St, Seattle" }
};
string CSVHeaderText = string.Join(SeperatorCharacter, CSVHeaderTextArray); // join the header text with seperator
//create and write data into the CSV file
try
{
// Using StreamWriter to create and write to a file
using (StreamWriter fwriter = new StreamWriter(CSVFileName, true)) //true opens the file in append mode
{
fwriter.WriteLine(CSVHeaderText); // write the header text to file
// Write
for (int i = 0; i < CSVDataArray.GetLength(0); i++)
{
StringBuilder sb = new StringBuilder();
for (int j = 0; j < CSVDataArray.GetLength(1); j++)
{
sb.Append(CSVDataArray[i, j]);
sb.Append(SeperatorCharacter);
}
sb.Length--; //remove the trailing comma
fwriter.WriteLine(sb.ToString()); //Write data to file
//Console.WriteLine(sb.ToString());
}//end of internal for loop
}//end of using
}//end of try
catch (Exception Ex)
{
Console.WriteLine($"An error occurred: {Ex.Message}");
}
}
}
}
All the parameters that are needed to control the creation of the CSV file are shown below.
//CSVfile creation parameters
string SeperatorCharacter = ","; // Comma Seperated file
string CSVFileName = "CSVTextFile.csv"; // Name of the file to be created
string[] CSVHeaderTextArray = { "No", "Name", "Age", "Address" }; //CSV header data
//CSV data to be stored
string[,] CSVDataArray = {
{ "1", "Alice", "30", "123 Main St, New York" },
{ "2", "Bob", "25", "456 Elm St, Los Angeles" },
{ "3", "Charlie", "35", "789 Oak St, Chicago" },
{ "4", "David", "40", "321 Pine St, Houston" },
{ "5", "Eve", "28", "654 Maple St, Seattle" }
};
Selecting the Delimiter for your CSV File
SeperatorCharacter variable is used to select the delimiter like a comma, colon, semicolon or the pipe symbol | .By changing this variable, you can get a comma delimited file ,colon delimited file or a tab delimited file as shown below.
Creating the header for your CSV file
All CSV files have a header that defines and describes the columns of the CSV file. The header values are also separated by the delimiter as shown above.
Here Array CSVHeaderTextArray is used to store the header data. You can change the header by either adding more headers or less depending upon your needs.
string[] CSVHeaderTextArray = { "No", "Name", "Age", "Address" }; //CSV header data
We then use the string.Join() method to join the header values with the delimiter. For Eg No, Name, Age, Address format inside the string CSVHeaderText
string CSVHeaderText = string.Join(SeperatorCharacter, CSVHeaderTextArray); // join the header text with seperator
// No,Name,Age,Address
Adding Data to your CSV file
All the data we need to write to our file is stored inside the 2 dimensional array CSVDataArray in No, Name, Age, Address format, this is done for convenience.
In real world applications, data will be provided by a separate file ,a network interface ,a database or a USB/Serial data acquisition system.
//CSV data to be stored
string[,] CSVDataArray = {
{ "1", "Alice", "30", "123 Main St, New York" },
{ "2", "Bob", "25", "456 Elm St, Los Angeles" },
{ "3", "Charlie", "35", "789 Oak St, Chicago" },
{ "4", "David", "40", "321 Pine St, Houston" },
{ "5", "Eve", "28", "654 Maple St, Seattle" }
};
Writing the data in CSV format
Now we will write the data from the array to a CSV text file in comma delimited format using C#. First thing we will do is to create the CSV text file on the disk using the name stored in the string variable CSVFileName.
string CSVFileName = "CSVTextFile.csv"; // Name of the file to be created
here we will use the StreamWriter class to create the file of specific name on the hard disk. If the file do not exist on the disk it will be created. If it exists, it will be opened in append mode (true) preserving the original data.
true parameter is only required if you want to append the data.
using (StreamWriter fwriter = new StreamWriter(CSVFileName, true)) //true opens the file in append mode
We will then write the header to our csv file and start appending data from 2d array CSVDataArray to our CSV text file. The whole code is also enclosed inside a try catch block.
//create and write data into the CSV file
try
{
// Using StreamWriter to create and write to a file
using (StreamWriter fwriter = new StreamWriter(CSVFileName, true)) //true opens the file in append mode
{
fwriter.WriteLine(CSVHeaderText); // write the header text to file
// Write
for (int i = 0; i < CSVDataArray.GetLength(0); i++)
{
StringBuilder sb = new StringBuilder();
for (int j = 0; j < CSVDataArray.GetLength(1); j++)
{
sb.Append(CSVDataArray[i, j]);
sb.Append(SeperatorCharacter);
}
sb.Length--; //remove the trailing comma
fwriter.WriteLine(sb.ToString()); //Write data to file
//Console.WriteLine(sb.ToString());
}//end of internal for loop
}//end of using
}//end of try
Here we are using two for loops to read the data from the 2d array and append them to the StringBuilder object sb along with the separator character( here comma) .
sb.Length--; // remove the trailing comma at the end
// 1,Alice,30,123 Main St, New York,<- trailing comma
The above line is used to remove the trailing comma at the end of the line.
finally the string is written line by line using the
fwriter.WriteLine(sb.ToString()); //Write data to file
Reading Data from CSV file using C#
Here we can use the StreamReader class to read the data stored in the CSV file line by line and then use the Split() method to seperate them to individual strings for further processing.
//Read data from CSV file
//full code in github,link above
try
{
using (StreamReader CSVReader = new StreamReader(CSVFileName))
{
string line;
while ((line = CSVReader.ReadLine()) != null) //readline by line till u meet the null char
{
Console.WriteLine(line);
string[] individualchar = line.Split(","); //split the line along comma
foreach(string c in individualchar)
{
Console.Write($"{c} ");
}
Console.WriteLine();
}
}
}
here is the output from the program.
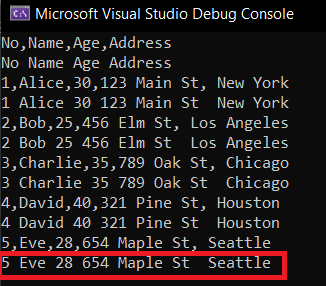
Creating a TAB separated Values File
You can create a TAB separated values file using our C# program by changing the SeperatorCharacter variable to a TAB (\t) character as shown below.
string SeperatorCharacter = "\t"; //create a TAB separated file using C#
on running this program it will create a TAB separated file on the disk.
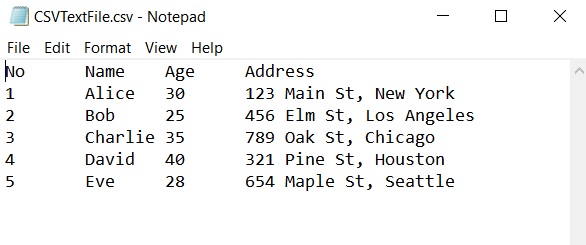
- Log in to post comments